How to execute one Controller Action from another in ASP.NET 5
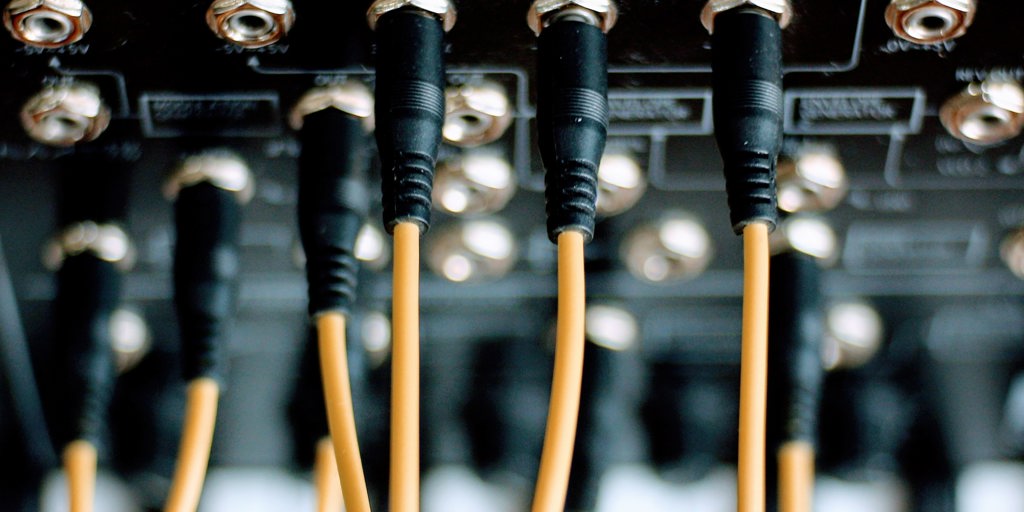
This will generally be a rare thing to do but if you have your reasons to do it, then this is how…
In Umbraco one valid reason to do this is due to how HTTP POSTs are handled for forms. Traditionally an HTML form will POST to a specific endpoint, that endpoint will handle the validation (etc), and if all is successful it will redirect to another URL, else it will return a validation result on the current URL (i.e. PRG POST/REDIRECT/GET). In the CMS world this may end up a little bit weird because URLs are dynamic. POSTs in theory should just POST to the current URL so that if there is a validation result, this is still shown on the current URL and not a custom controller endpoint URL. This means that there can be multiple controllers handling the same URL, one for GET, another for POST and that’s exactly what Umbraco has been doing since MVC was enabled in it many years ago. For this to work, a controller is selected during the dynamic route to handle the POST (a SurfaceController in Umbraco) and if successful, typically the developer will use: return RedirectToCurrentUmbracoPage (of type RedirectToUmbracoPageResult) or if not successful will use: return CurrentUmbracoPage (of type UmbracoPageResult). The RedirectToUmbracoPageResult is easy to handle since this is just a redirect but the UmbracoPageResult is a little tricky because one controller has just handled the POST request but now it wants to return a page result for the current Umbraco page which is handled by a different controller.
IActionInvoker
The concept is actually pretty simple and the IActionInvoker does all of the work. You can create an IActionInvoker from the IActionInvokerFactory which needs an ActionContext. Here’s what the ExecuteResultAsync method of a custom IActionResult could look like to do this:
public async Task ExecuteResultAsync(ActionContext context) { // Change the route values to match the action to be executed context.RouteData.Values["controller"] = "Page"; context.RouteData.Values["action"] = "Index"; // Create a new context and excute the controller/action // Copy the action context - this also copies the ModelState var renderActionContext = new ActionContext(context) { // Normally this would be looked up via the EndpointDataSource // or using the IActionSelector ActionDescriptor = new ControllerActionDescriptor { ActionName = "Index", ControllerName = "Page", ControllerTypeInfo = typeof(PageController).GetTypeInfo(), DisplayName = "PageController.Index" } }; // Get the factory IActionInvokerFactory actionInvokerFactory = context.HttpContext .RequestServices .GetRequiredService<IActionInvokerFactory>(); // Create the invoker IActionInvoker actionInvoker = actionInvokerFactory.CreateInvoker(renderActionContext); // Execute! await actionInvoker.InvokeAsync(); }
That’s pretty must the gist of it. The note about the ControllerActionDescriptor is important though, it’s probably best to not manually create these since they are already created with all of your routing. They can be queried and resolved in a few different ways such as interrogating the EndpointDataSource or using the IActionSelector. This execution will execute the entire pipeline for the other controller including all of it’s filters, etc…