Smidge 2.0 alpha is out
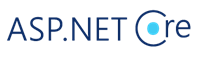
What is Smidge? Smidge is a lightweight runtime bundling library (CSS/JavaScript file minification, combination, compression) for ASP.NET Core.
If you’ve come from ASP.NET 4.5 you would have been familiar with the bundling/minification API and other bundling options like ClientDependency, but that is no longer available in ASP.NET Core, instead it is advised to do all the bundling and pre-processing that you need as part of your build process …which certainly makes sense! So why create this library? A few reasons: some people just want to have a very simple bundling library and don’t want to worry about Gulp or Grunt or WebPack, in a lot of cases the overhead of runtime processing is not going to make any difference, and lastly, if you have created something like a CMS that dynamically loads in assets from 3rd party packages or plugins, you need a runtime bundler since these things don’t exist at build time.
Over the past few months I’ve been working on some enhancements to Smidge and have found a bit of time to get an alpha released. There’s loads of great new features in Smidge 2.0! You can install via Nuget and is targets .NET Standard 1.6 and .NET Framework 4.5.2
PM> Install-Package Smidge -Pre
New to Smidge?
It’s easy to get started with Smidge and there’s lots of docs available on GitHub that cover installation, configuration, creating bundles and rendering them.
New Features
Here’s a list of new features complete with lots of code examples
Customizable Debug and Production options
https://github.com/Shazwazza/Smidge/issues/58
Previous to version 2.0, you could only configure aspects of the Production options and the Debug assets that were returned were just the raw static files. With 2.0, you have full control over how your assets are processed in both Debug and Production configurations. For example, if you wanted you could have your assets combined but not minified in Debug mode. This will also allow for non native web assets such as TypeScript to have pre-processors running and able to work in Debug mode.
Example:
services.AddSmidge(_config) .Configure<SmidgeOptions>(options => { //set the default e-tag options for Debug mode options.DefaultBundleOptions.DebugOptions.CacheControlOptions.EnableETag = false });
Fluent syntax for declaring/configuring bundles
https://github.com/Shazwazza/Smidge/issues/55
If you want to customize Debug or Production options per bundle, you can do so with a fluent syntax, for example:
app.UseSmidge(bundles => { //For this bundle, enable composite files for Debug mode, enable the file watcher so any changes //to the files are automatically re-processed and cache invalidated, disable cache control headers //and use a custom cache buster. You could of course use the .ForProduction options too bundles.Create("test-bundle-2", WebFileType.Js, "~/Js/Bundle2") .WithEnvironmentOptions(BundleEnvironmentOptions.Create() .ForDebug(builder => builder .EnableCompositeProcessing() .EnableFileWatcher() .SetCacheBusterType<AppDomainLifetimeCacheBuster>() .CacheControlOptions(enableEtag: false, cacheControlMaxAge: 0)) .Build() ); });
Customizable Cache Buster
https://github.com/Shazwazza/Smidge/issues/51
In version 1.0 the only cache busting mechanism was Smidge’s version property which is set in config, in 2.0 Smidge allows you to control how cache busting is controlled at a global and bundle level. 2.0 ships with 2 ICacheBuster types:
-
ConfigCacheBuster – the default and uses Smidge’s version property in config
-
AppDomainLifetimeCacheBuster – if enabled will mean that the server/browser cache will be invalidated on every app domain recycle
If you want a different behavior, you can define you own ICacheBuster add it to the IoC container and then just use it globally or per bundle. For example:
//Set a custom MyCacheBuster as the default one for Debug assets: services.AddSmidge(_config) .Configure<SmidgeOptions>(options => { options.DefaultBundleOptions.DebugOptions.SetCacheBusterType<MyCustomCacheBuster>(); }); //Set a custom MyCacheBuster as the cache buster for a particular bundle in debug mode: bundles.Create("test-bundle-2", WebFileType.Js, "~/Js/Bundle2") .WithEnvironmentOptions(BundleEnvironmentOptions.Create() .ForDebug(builder => builder .SetCacheBusterType<MyCacheBuster>() .Build() );
Customizable cache headers
https://github.com/Shazwazza/Smidge/issues/48
You can now control if you want the ETag header output and you can control the value set for max-age/s-maxage/Expires header at a global or bundle level, for example:
//This would set the max-age header for this bundle to expire in 5 days bundles.Create("test-bundle-5", WebFileType.Js, "~/Js/Bundle5") .WithEnvironmentOptions(BundleEnvironmentOptions.Create() .ForProduction(builder => builder .CacheControlOptions(enableEtag: true, cacheControlMaxAge: (5 * 24))) .Build() );
Callback to customize the pre-processor pipeline per web file
https://github.com/Shazwazza/Smidge/issues/59
This is handy in case you want to modify the pipeline for a given web file at runtime based on some criteria, for example:
services.AddSmidge(_config) .Configure<SmidgeOptions>(options => { //set the callback options.PipelineFactory.OnGetDefault = GetDefaultPipelineFactory; }); //The GetDefaultPipeline method could do something like modify the default pipeline to use Nuglify for JS processing: private static PreProcessPipeline GetDefaultPipelineFactory(WebFileType fileType, IReadOnlyCollection<IPreProcessor> processors) { switch (fileType) { case WebFileType.Js: return new PreProcessPipeline(new IPreProcessor[] { processors.OfType<NuglifyJs>().Single() }); } //returning null will fallback to the logic defined in the registered PreProcessPipelineFactory return null; }
File watching with automatic cache invalidation
https://github.com/Shazwazza/Smidge/pull/42
During the development process it would be nice to be able to test composite files but have them auto re-process and invalidate the cache whenever one of the source files changes… in 2.0 this is possible! You can enable file watching at the global level or per bundle. Example:
//Enable file watching for all files in this bundle when in Debug mode bundles.Create("test-bundle-7", new CssFile("~/Js/Bundle7/a1.js"), new CssFile("~/Js/Bundle7/a2.js")) .WithEnvironmentOptions(BundleEnvironmentOptions.Create() .ForDebug(builder => builder.EnableFileWatcher()) .Build() );
What’s next?
This is an alpha release since there’s a few things that I need to complete. Most are already done but I just need to make Nuget packages for them:
More pre-processors
I’ve enabled support for a Nuglify pre-processor for both CSS and JS (Nuglify is a fork of the Microsoft Ajax Minifier for ASP.NET Core + additional features). I also enabled support for an Uglify NodeJs pre-processor which uses Microsoft.AspNetCore.NodeServices to invoke Node.js from ASP.NET and run the JS version of Uglify. I just need to get these on Nuget but haven’t got around to that yet.
A quick note on minifier performance
Though Nuglify and Uglify have a better minification engine (better/smarter size reduction) than JsMin because they create an AST (Abstract Syntax Tree) to perform it’s processing, they are actually much slower and consume more resources than JsMin. Since Smidge is a Runtime bundling engine, its generally important to ensure that the bundling/minification is performed quickly. Smidge has strict caching so the bundling/minification will only happen once (depending on your ICacheBuster you are using) but it is still recommended to understand the performance implications of replacing JsMin with another minifier. I’ve put together some benchmarks (NOTE: a smaller Minified % is better):
Method | Median | StdDev | Scaled | Scaled-SD | Minified % | Gen 0 | Gen 1 | Gen 2 | Bytes Allocated/Op |
---|---|---|---|---|---|---|---|---|---|
JsMin | 10.2008 ms | 0.3102 ms | 1.00 | 0.00 | 51.75% | - | - | - | 155,624.67 |
Nuglify | 69.0778 ms | 0.0180 ms | 6.72 | 0.16 | 32.71% | 53.00 | 22.00 | 15.00 | 4,837,313.07 |
JsServicesUglify | 1,548.3951 ms | 7.6388 ms | 150.95 | 3.73 | 32.63% | 0.97 | - | - | 576,056.55 |
Thanks!
Big thanks to @dazinator for all the help, recommendations, testing, feedback, etc… and for the rest of the community for filing bugs, questions, and comments. Much appreciated :)