Need to remove an auto-routed controller in Umbraco?
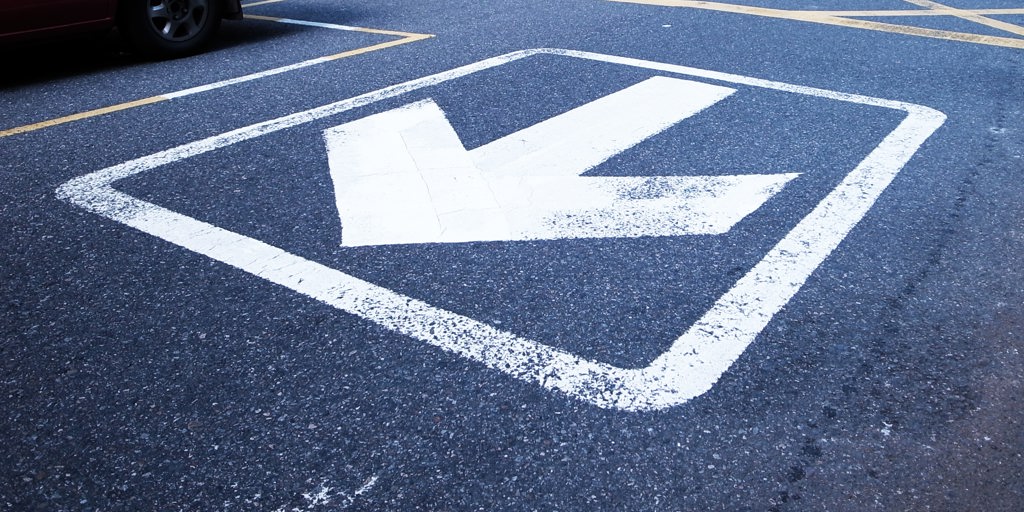
Umbraco will auto-route some controllers automatically. These controllers are any MVC SurfaceControllers or WebApi UmbracoApiController types discovered during startup. There might be some cases where you just don’t want these controllers to be routed at all, maybe a package installs a controller that you’d rather not have routable or maybe you want to control if your own plugin controllers are auto-routed based on some configuration.
The good news is that this is quite easy by just removing these routes during startup. There’s various ways you could do this but I’ve shown below one of the ways to interrogate the routes that have been created to remove the ones you don’t want:
Version 8
//This is required to ensure this composer runs after
//Umbraco's WebFinalComposer which is the component
//that creates all of the routes during startup
[ComposeAfter(typeof(WebFinalComposer))]
public class MyComposer : ComponentComposer<MyComponent>{ }
//The component that runs after WebFinalComponent
//during startup to modify the routes
public class MyComponent : IComponent
{
public void Initialize()
{
//list the routes you want removed, in this example
//this will remove the built in Umbraco UmbRegisterController
//and the TagsController from being routed.
var removeRoutes = new[]
{
"/surface/umbregister",
"/api/tags"
};
foreach (var route in RouteTable.Routes.OfType().ToList())
{
if (removeRoutes.Any(r => route.Url.InvariantContains(r)))
RouteTable.Routes.Remove(route);
}
}
public void Terminate() { }
}
Version 7
public class MyStartupHandler : ApplicationEventHandler
{
protected override void ApplicationStarted(
UmbracoApplicationBase umbracoApplication,
ApplicationContext applicationContext)
{
//list the routes you want removed, in this example
//this will remove the built in Umbraco UmbRegisterController
//and the TagsController from being routed.
var removeRoutes = new[]
{
"/surface/umbregister",
"/api/tags"
};
foreach(var route in RouteTable.Routes.OfType<Route>().ToList())
{
if (removeRoutes.Any(r => route.Url.InvariantContains(r)))
RouteTable.Routes.Remove(route);
}
}
}
comments powered by Disqus